https://www.acmicpc.net/problem/11279
11279번: 최대 힙
첫째 줄에 연산의 개수 N(1 ≤ N ≤ 100,000)이 주어진다. 다음 N개의 줄에는 연산에 대한 정보를 나타내는 정수 x가 주어진다. 만약 x가 자연수라면 배열에 x라는 값을 넣는(추가하는) 연산이고, x가
www.acmicpc.net
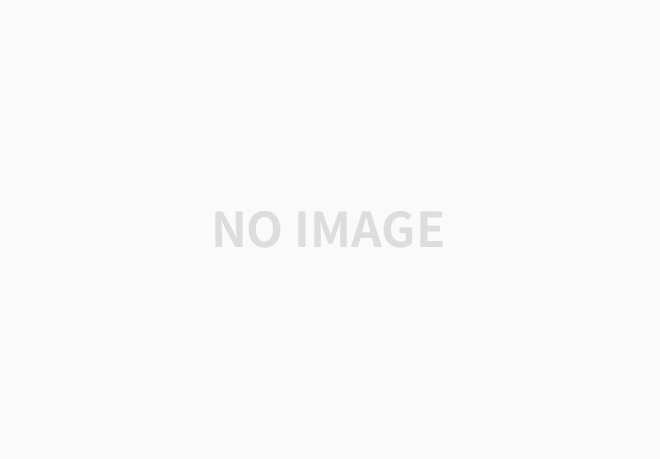
우선순위 큐
시간제한이 칼같길래..힙 구현해서 풀어야하는줄알고 오랜만에 힙을 다시 공부했는데
STL로 그냥 풀리는 문제였다..기왕한거 최대힙을 구현해서 풀어봤다.
오답노트
시간초과가 한번 났는데 cin tie 끊고 sync_with_stdio false로 하니 풀렸다.
소스코드
priority_queue STL version (AC)
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
int main(){
ios::sync_with_stdio(false);
cin.tie(NULL);
priority_queue<int, vector<int>, less<int>> heap;
int t;
cin >> t;
int x;
for(int i = 0 ; i < t ; i++){
cin >> x;
if(x == 0){
if(heap.empty()){
cout << "0\n";
}else{
cout << heap.top() << "\n";
heap.pop();
}
}else{
heap.push(x);
}
}
return 0;
}
max heap 구현 version (not AC)
#include <iostream>
using namespace std;
int heap[100001];
int heapCount = -1;
void push(int n){
heap[++heapCount] = n;
int child = heapCount;
int parent = child/2;
while(child > 0 && heap[child] > heap[parent]){
int temp = heap[child];
heap[child] = heap[parent];
heap[parent] = temp;
child = parent;
parent = child/2;
}
}
void print(){
for(int i = 0 ; i <= heapCount ; i++){
cout << heap[i] << endl;
}
}
int pop(){
int top = heap[0];
heap[0] = heap[heapCount--];
int parent = 0;
int child = 1;
if(child+1 <= heapCount)
child = (heap[child]>heap[child+1]) ? child : child+1;
while(parent <= heapCount && heap[parent] < heap[child]) {
int temp = heap[parent];
heap[parent] = heap[child];
heap[child] = temp;
parent = child;
child = parent*2+1;
if(child+1 <= heapCount)
child = (heap[child]>heap[child+1]) ? child : child+1;
}
return top;
}
int main(){
ios::sync_with_stdio(false);
cin.tie(NULL);
int t;
cin >> t;
int x;
for(int i = 0 ; i < t ; i++){
cin >> x;
if(x == 0){
if(heapCount == -1){
cout << "0\n";
}else{
cout << pop() << "\n";
}
}else{
push(x);
}
}
return 0;
}
728x90
'알고리즘 문제풀이' 카테고리의 다른 글
[백준 11286] 알고리즘 97일차 : 절댓값 힙 (0) | 2021.07.01 |
---|---|
[백준 1927] 알고리즘 96일차 : 최소 힙 (0) | 2021.06.30 |
[백준 11066] 알고리즘 94일차 : 파일 합치기 (0) | 2021.06.28 |
[프로그래머스] 튜플 (0) | 2021.05.07 |
[프로그래머스] 수식최대화 (0) | 2021.05.07 |